Algorithms
The sequence of steps to be performed in order to solve a problem
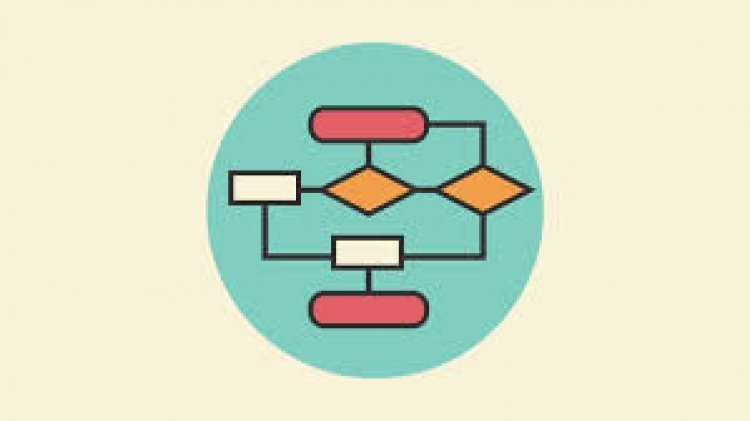
Hello everyone,
A computer is a useful tool for solving a variety of problems. To make a computer do any task, you have to write a computer program. In a computer program, you have to tell a computer, step by step, what you want it to do exactly. Then the computer executes the program, following each step mechanically, to accomplish its end goal. A popular tool and technique used to represent the programs is an algorithm.
ALGORITHMS
An algorithm is a set of rules that defines how a particular problem can be solved in a finite number of steps to be written in simple English. In other words, an algorithm is a step-by-step representation of instructions to solve a problem. An algorithm must consist of a finite set of steps for which we may require one or more operations. An algorithm written in computer language constitutes a program.
Feature of an algorithm:
- Each step of the algorithm should be simple.
- No ambiguity should exist in an algorithm,i.e, the logic should be crisp and clear.
- It should be effective enough and provide a unique solution to the problem.
- It must execute and terminate in a finite number of steps.
- It should be efficient enough to perform a task in minimum lines of code.
Key Roles of Algorithm
- Input: This part of the algorithm accepts the data by fetching the input from the user for a given problem
- Process: This part of the algorithm does the required computations with the help of simple steps.
- Output: It must produce the expected outcome or output.
Properties of Algorithm
How to Develop an Algorithm
To develop an algorithm, the problem has to be analyzed properly. The programmer must understand the problem and come out with the most appropriate method or logic that can solve the problem. The following steps are to be taken for developing an algorithm:
- Understand the problem,
- Identify the output of the problem.
- Identify the inputs required by the problem to achieve the desired output.
- Design a logic for the problem for which it will produce the desired output from the given inputs.
- Test the algorithm for a given set of input data.
- Repeat steps 1 to 5 until the algorithm gives the desired results.
Let us take the example to understand the concept of algorithm.
Algorithm to swap values of two locations, P and Q:
Steps:
- Read the values of P and Q.
- Save the initial values of P in TEMP.
- Move the initial value of Q in P.
- Move TEMP into Q.
- Display the exchanged value of P and Q.
- Stop.
It's the most important topic to get started with programming. More will come soon related to coding.